How to set a gradient background for the Container.
We will need the decoration
attribute in Container.
LinearGradient is used by BoxDecoration to represent linear gradients.
A gradient has two anchor points, begin
and end
. The begin
point corresponds to 0.0, and the end
point corresponds to 1.0
decoration: const BoxDecoration( gradient: LinearGradient( begin: Alignment.topRight, end: Alignment.bottomLeft, colors: [Color(0xFF4C4C7B), Color(0xFF741826)])),
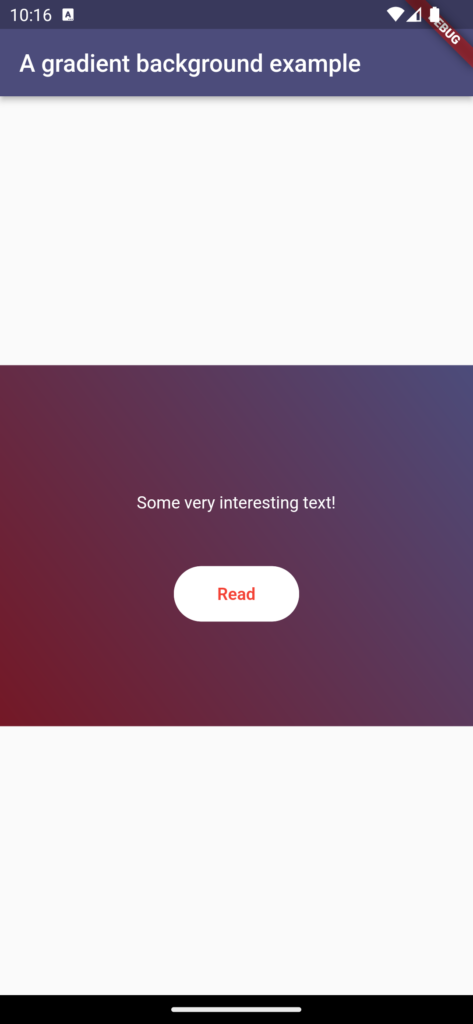
Full code:
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return const MaterialApp( title: 'Flutter Demo', home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key}); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { Size size = MediaQuery.of(context).size; return Scaffold( appBar: AppBar( backgroundColor: const Color(0xFF4C4C7B), title: const Text('A gradient background example'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Container( decoration: const BoxDecoration( gradient: LinearGradient( begin: Alignment.topRight, end: Alignment.bottomLeft, colors: [Color(0xFF4C4C7B), Color(0xFF741826)])), height: 300, width: MediaQuery.of(context).size.width, child: Column( crossAxisAlignment: CrossAxisAlignment.center, mainAxisAlignment: MainAxisAlignment.center, children: [ Row( mainAxisAlignment: MainAxisAlignment.center, children: [ SizedBox( height: 80, width: size.width * 0.90, child: const Padding( padding: EdgeInsets.fromLTRB(0, 20, 0, 20), child: Text( 'Some very interesting text!', textAlign: TextAlign.center, style: TextStyle( fontSize: 14, color: Colors.white, fontFamily: 'Open-Sans', ), ), ), ) ], ), Row( mainAxisAlignment: MainAxisAlignment.center, children: [ OutlinedButton( style: ButtonStyle( backgroundColor: MaterialStateProperty.all(Colors.white), side: MaterialStateProperty.all(const BorderSide( width: 0.5, color: Colors.white)), shape: MaterialStateProperty.all( RoundedRectangleBorder( borderRadius: BorderRadius.circular(35.0))), ), onPressed: () {}, child: const Padding( padding: EdgeInsets.fromLTRB(20.0, 15, 20, 15), child: Text( 'Read', style: TextStyle( color: Colors.red, fontWeight: FontWeight.w600, fontSize: 14), ), )) ], ) ], ), ) ], ), ), ); } }