How to add the Modal Bottom Sheet.
We can use the embedded function showModalBottomSheet
.
A modal bottom sheet is an alternative to a menu or a dialog and prevents the user from interacting with the rest of the app.
How it looks:
Future<T?> showModalBottomSheet<T>( {required BuildContext context, required WidgetBuilder builder, Color? backgroundColor, double? elevation, ShapeBorder? shape, Clip? clipBehavior, BoxConstraints? constraints, Color? barrierColor, bool isScrollControlled = false, bool useRootNavigator = false, bool isDismissible = true, bool enableDrag = true, bool useSafeArea = false, RouteSettings? routeSettings, AnimationController? transitionAnimationController, Offset? anchorPoint} )
It has two required properties – context
and builder
The context
argument is used to look up the Navigator
and Theme
for the bottom sheet.
The builder
is a function that takes the current context and returns a widget.
How to create a modal bottom sheet in Flutter:
All we need is just to add this piece of code in the required place:
showModalBottomSheet( context: context, builder: (BuildContext context) { return Container(); });
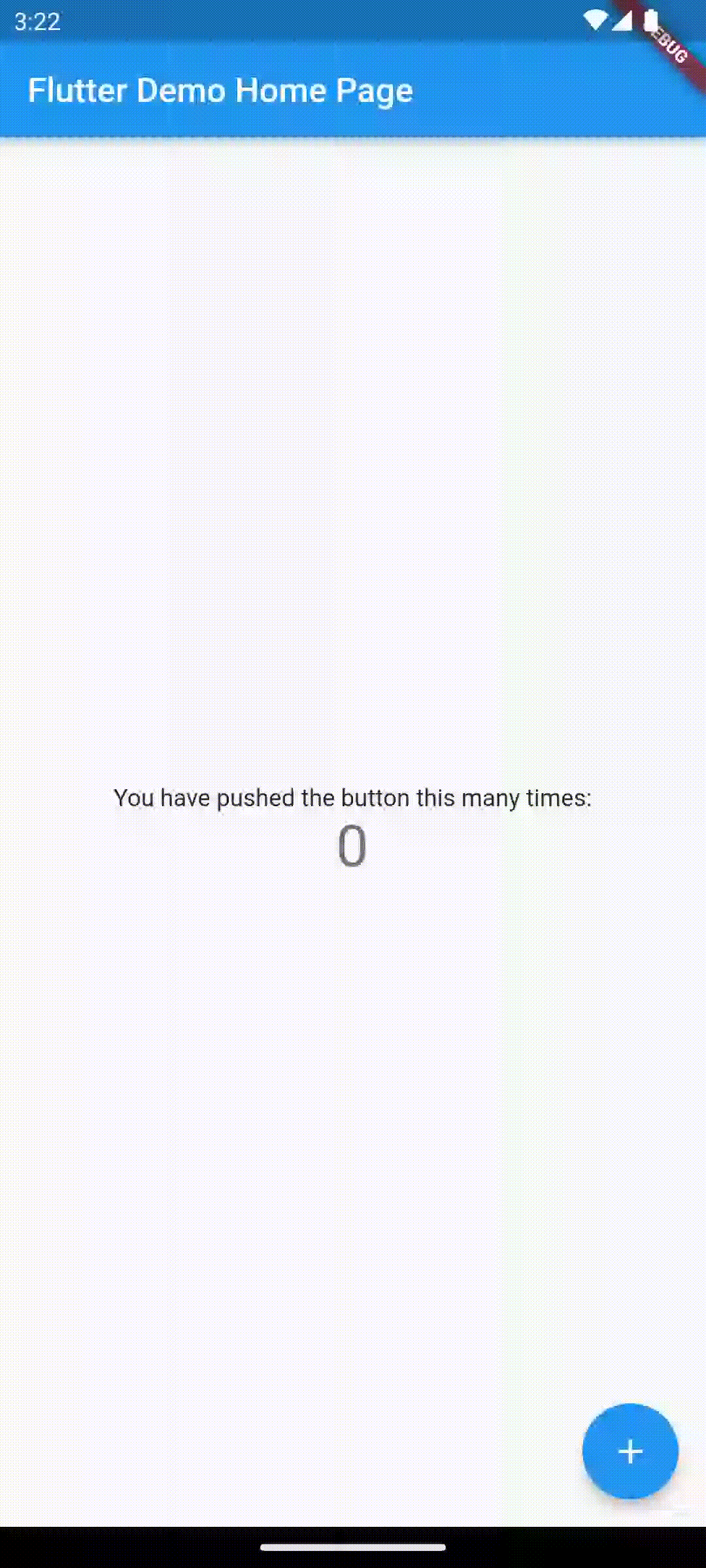
Full code of example:
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(title: 'Flutter Demo Home Page'), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key, required this.title}); final String title; @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { int _counter = 0; void increment() { setState(() { _counter = _counter + 1; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ const Text( 'You have pushed the button this many times:', ), Text( '$_counter', style: Theme.of(context).textTheme.headline4, ), ], ), ), floatingActionButton: FloatingActionButton( onPressed: () { showModalBottomSheet( context: context, builder: (BuildContext context) { return Container( child: SizedBox( height: 150, child: Column( children: [ Row( crossAxisAlignment: CrossAxisAlignment.center, mainAxisAlignment: MainAxisAlignment.center, children: [ SizedBox( width: MediaQuery.of(context).size.width, child: OutlinedButton( style: OutlinedButton.styleFrom( side: BorderSide.none, ), onPressed: () { increment(); Navigator.pop(context); }, child: const Text('Increment'), ), ), ], ), Row( crossAxisAlignment: CrossAxisAlignment.center, mainAxisAlignment: MainAxisAlignment.center, children: [ SizedBox( width: MediaQuery.of(context).size.width, child: OutlinedButton( style: OutlinedButton.styleFrom( side: BorderSide.none, ), onPressed: () { Navigator.pop(context); }, child: const Text('Cancel'), ), ), ], ) ], ), ), ); }); }, tooltip: 'Increment', child: const Icon(Icons.add), ), // This trailing comma makes auto-formatting nicer for build methods. ); } }
Read more information here – showModalBottomSheet