This example shows you how to add a custom font in Flutter.
To display a string in flutter we use Text
widget.
Text
– displays a string of text with single style. The string might break across multiple lines or might all be displayed on the same line depending on the layout constraints.
Let’s check the constructor of Text
widget:
Text( String data, { Key? key, TextStyle? style, StrutStyle? strutStyle, TextAlign? textAlign, TextDirection? textDirection, Locale? locale, bool? softWrap, TextOverflow? overflow, double? textScaleFactor, int? maxLines, String? semanticsLabel, TextWidthBasis? textWidthBasis, TextHeightBehavior? textHeightBehavior, Color? selectionColor } )
We need the style
parameter. You can read more about TextStyle here.
TextStyle
– an immutable style describing how to format and paint text.
To find some fonts you can here.
First of all, you need to add your font file to flutter project.
1) Create directories “assets/font
” in your project and copy your font inside.
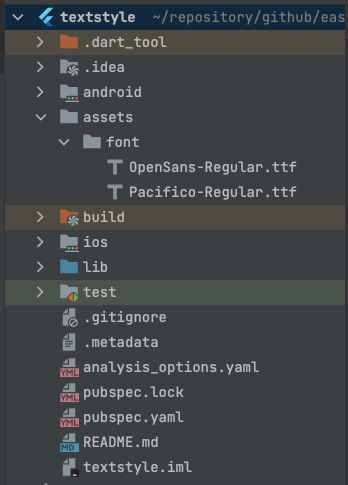
2) Add your font to pubspec.yaml
Inside pubspec.yaml
we need fonts
section.
name: textstyle description: A TextStyle examples publish_to: 'none' version: 1.0.0+1 environment: sdk: '>=2.18.5 <3.0.0' dependencies: flutter: sdk: flutter cupertino_icons: ^1.0.2 dev_dependencies: flutter_test: sdk: flutter flutter_lints: ^2.0.0 flutter: uses-material-design: true fonts: - family: Pacifico-Regular fonts: - asset: assets/font/Pacifico-Regular.ttf - family: OpenSans fonts: - asset: assets/font/OpenSans-Regular.ttf
3) Add your font family to TextStyle parameter fontFamily
:
Text( 'Some amazing and meaningful text', style: TextStyle(fontSize: 18, fontFamily: 'OpenSans'), )
The result of the example:
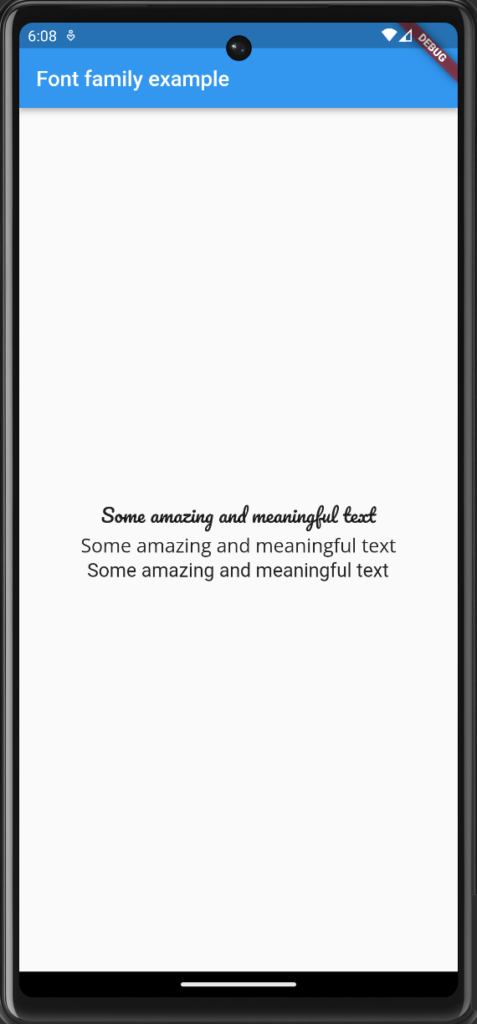
Full source code of example:
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(title: 'Font family example'), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key, required this.title}); final String title; @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Row( mainAxisAlignment: MainAxisAlignment.center, children: const [ Text( 'Some amazing and meaningful text', style: TextStyle(fontSize: 18, fontFamily: 'Pacifico-Regular'), ), ], ), Row( mainAxisAlignment: MainAxisAlignment.center, children: const [ Text( 'Some amazing and meaningful text', style: TextStyle(fontSize: 18, fontFamily: 'OpenSans'), ), ], ), Row( mainAxisAlignment: MainAxisAlignment.center, children: const [ Text( 'Some amazing and meaningful text', style: TextStyle( fontSize: 18, ), ), ], ), ], ), ), ); } }