In this post, we will explore how to create a custom animated button in Flutter. A custom animated button can greatly enhance the user experience by providing interactive and visually appealing feedback. We will build a button that changes its background color and text color when pressed, with a smooth animation effect.
Prerequisites
- Basic understanding of Flutter and Dart
- Flutter SDK installed and set up
Step 1: Create a new Flutter project
First, create a new Flutter project using the following command:
flutter create custom_animated_button
Then, navigate to the project directory:
cd custom_animated_button
Open the project in your favorite code editor.
Step 2: Create the CustomAnimatedButton widget
In the lib
folder, create a new file called custom_animated_button.dart
. Inside this file, define the CustomAnimatedButton
widget:
import 'package:flutter/material.dart'; class CustomAnimatedButton extends StatefulWidget { final String buttonText; final VoidCallback onPressed; const CustomAnimatedButton({required this.buttonText, required this.onPressed}); @override _CustomAnimatedButtonState createState() => _CustomAnimatedButtonState(); } class _CustomAnimatedButtonState extends State<CustomAnimatedButton> with SingleTickerProviderStateMixin { late AnimationController _animationController; late Animation<Color?> _backgroundColor; late Animation<Color?> _textColor; @override void initState() { super.initState(); _animationController = AnimationController( duration: const Duration(milliseconds: 200), vsync: this, ); _backgroundColor = ColorTween( begin: Colors.blue, end: Colors.red, ).animate(_animationController); _textColor = ColorTween( begin: Colors.white, end: Colors.black, ).animate(_animationController); } @override void dispose() { _animationController.dispose(); super.dispose(); } void _onButtonTap() { setState(() { if (_animationController.status == AnimationStatus.completed) { _animationController.reverse(); } else { _animationController.forward(); } }); widget.onPressed(); } @override Widget build(BuildContext context) { return AnimatedBuilder( animation: _animationController, builder: (context, child) { return MaterialButton( color: _backgroundColor.value, textColor: _textColor.value, child: child, onPressed: _onButtonTap, ); }, child: Text(widget.buttonText), ); } }
This widget is a StatefulWidget, as it needs to maintain the state of the animation. The button has two color animations, one for the background and one for the text. When the button is tapped, it will trigger the animation, and call the onPressed callback provided by the parent.
Step 3: Use the CustomAnimatedButton in the main application
In the lib/main.dart
file, replace the code with the following:
import 'package:flutter/material.dart'; import 'custom_animated_button.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Custom Animated Button', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatelessWidget { void _onButtonPressed() { print('Button Pressed'); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Custom Animated Button'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ CustomAnimatedButton( buttonText: 'Tap me!', onPressed: _onButtonPressed, ), ], ), ), ); } }
With this code, the MyHomePage
widget uses the CustomAnimatedButton
widget in the center of the screen. When the button is tapped, it will animate the background and text colors while also executing the _onButtonPressed
function, which simply prints “Button Pressed” to the console.
Now you can run the app using the following command:
flutter run
You should see the custom animated button in the center of the screen, and the color change animation will occur when the button is tapped. This is just a simple example, but you can customize and extend this widget to fit your specific needs, creating more complex animations or incorporating additional functionality.
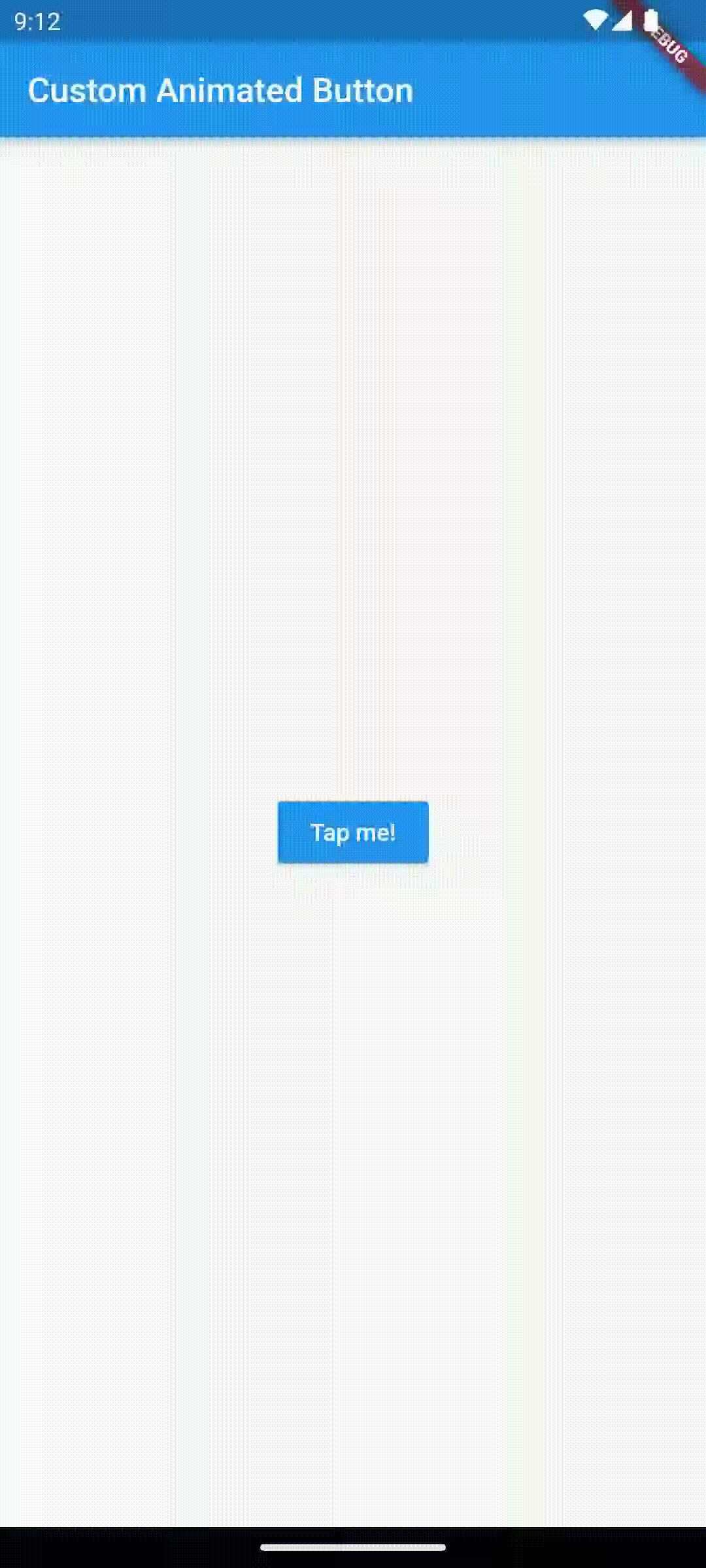