This example shows you how to set keyboard type on TextField
in Flutter.
TextField
has keyboardType
parameter.
TextField( keyboardType: , )
Name:
TextField( decoration: InputDecoration( labelText: 'Name' ), keyboardType: TextInputType.name, )
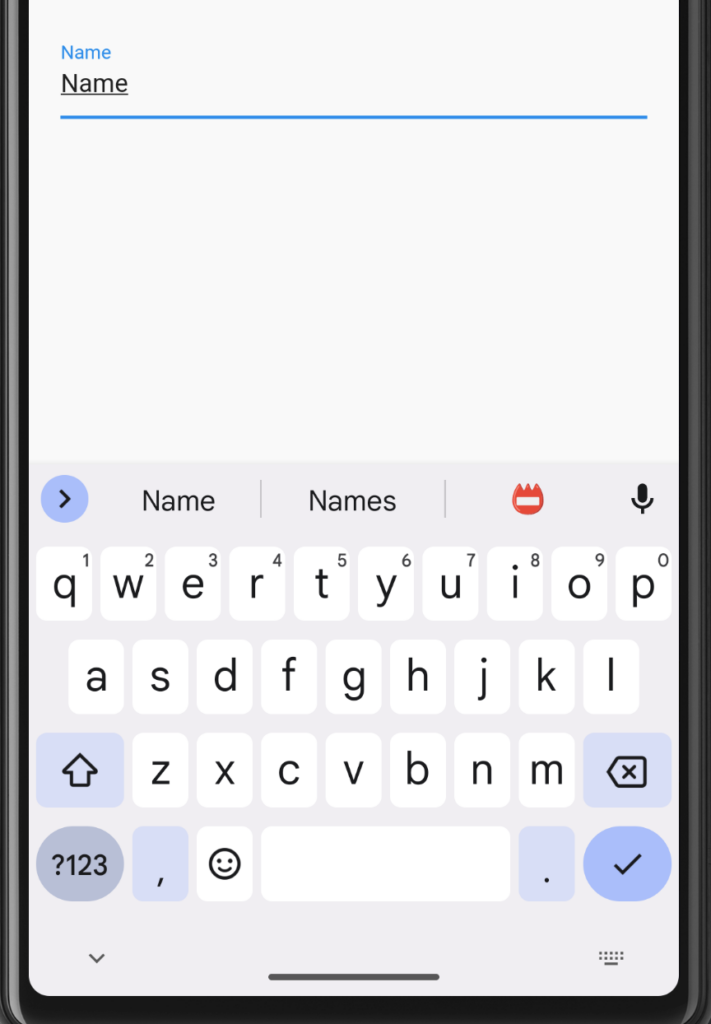
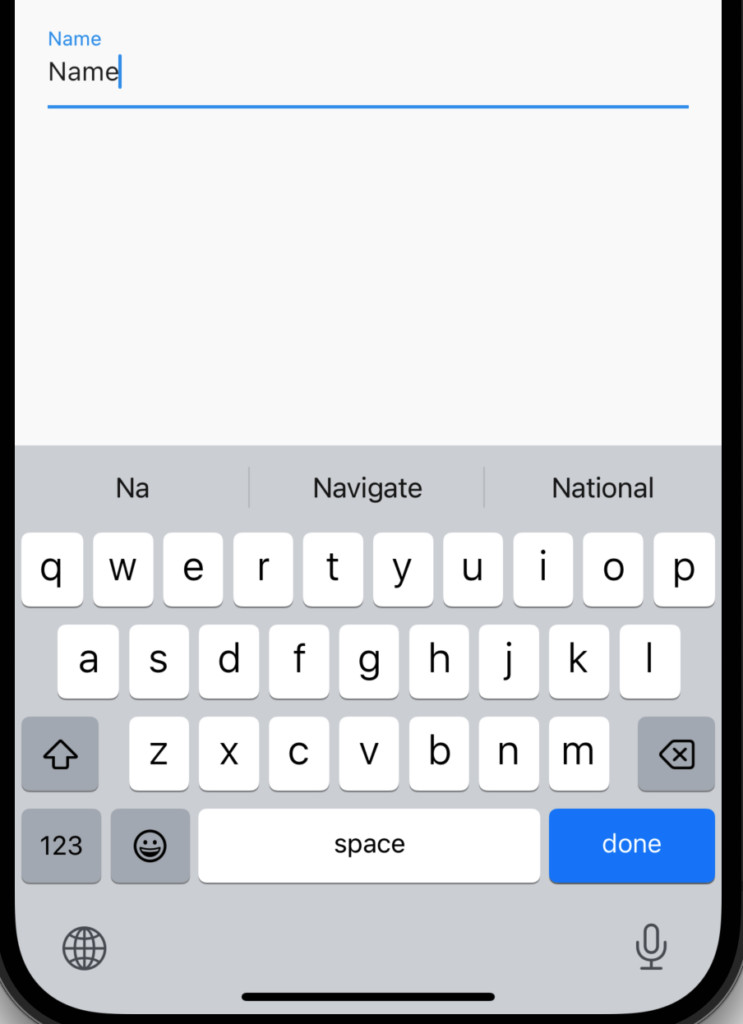
Email:
TextField( decoration: InputDecoration( labelText: 'Email' ), keyboardType: TextInputType.emailAddress, )
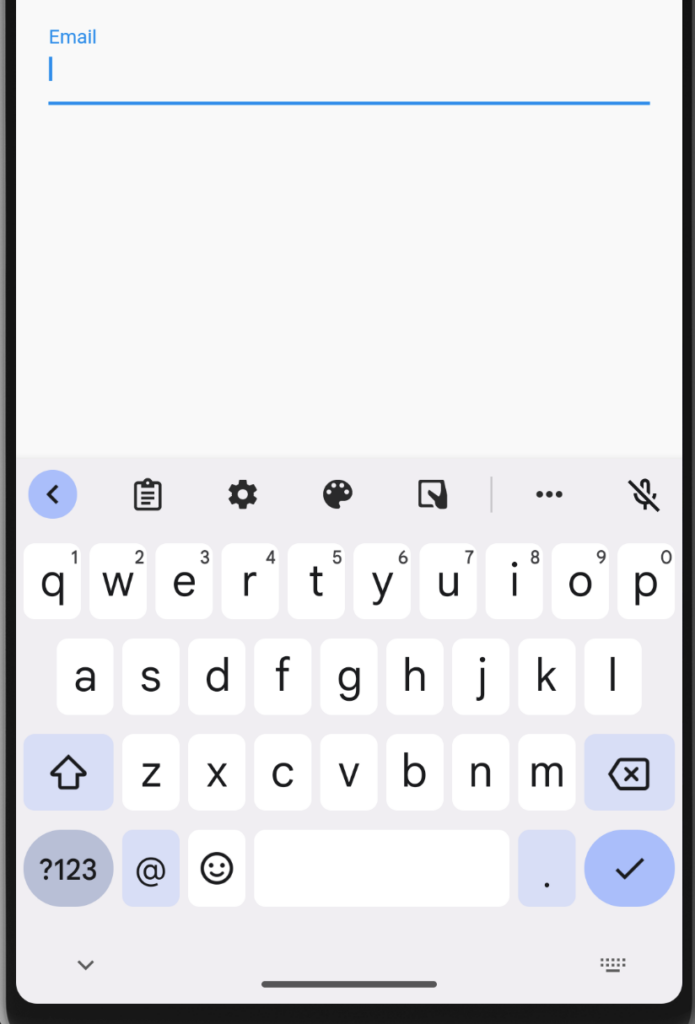
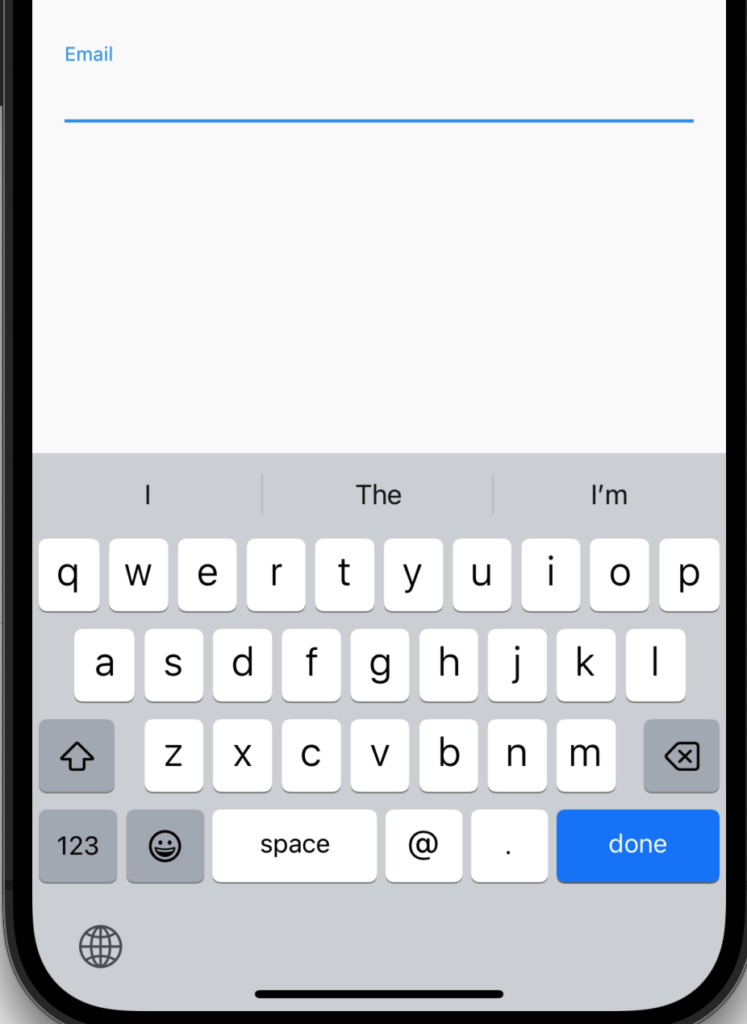
Phone number:
TextField( decoration: InputDecoration( labelText: 'Phone number' ), keyboardType: TextInputType.phone, )
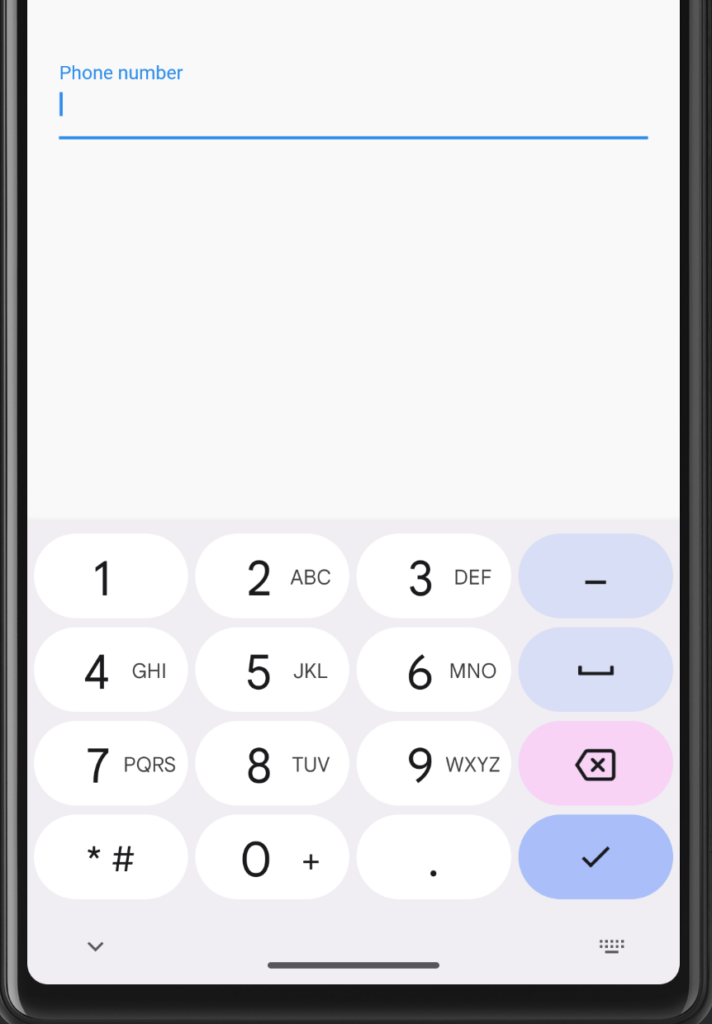
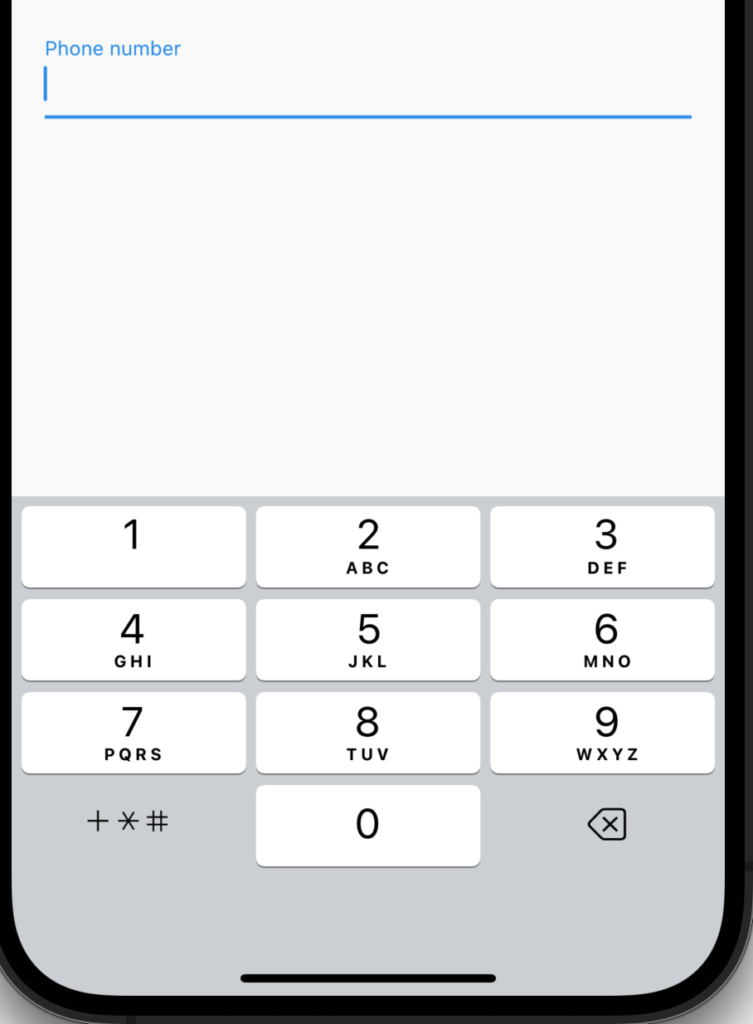
Datetime:
TextField( decoration: InputDecoration( labelText: 'Datetime' ), keyboardType: TextInputType.datetime, )
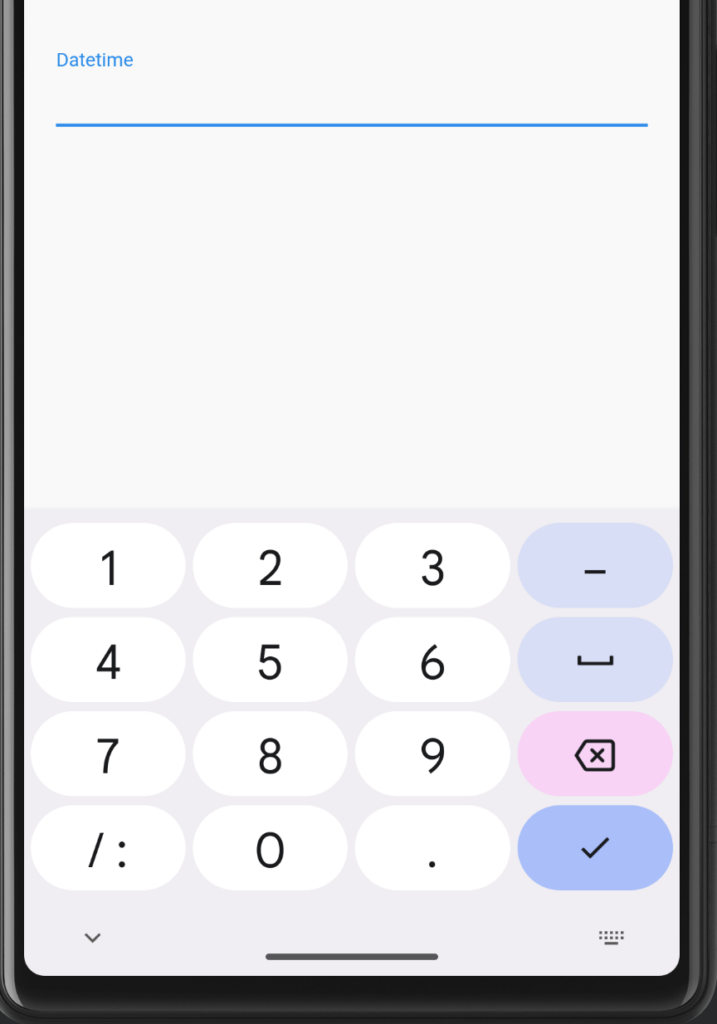
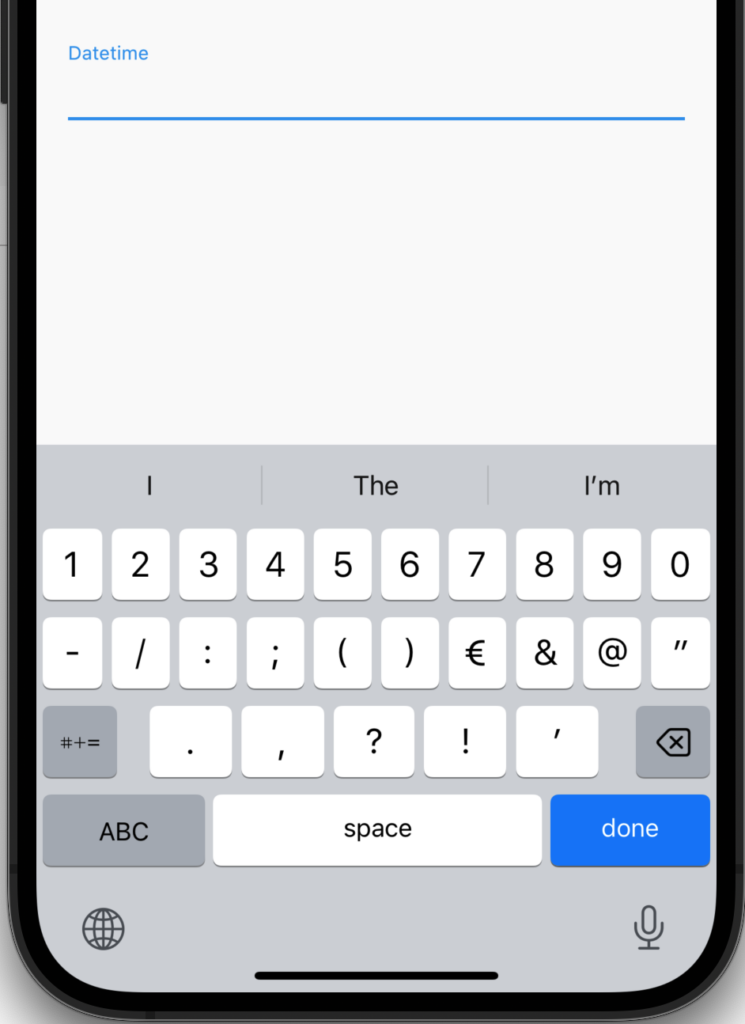
Full source code:
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(title: 'Flutter Demo Home Page'), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key, required this.title}); final String title; @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Padding( padding: const EdgeInsets.all(20), child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Row( children: const [ Expanded( child: TextField( decoration: InputDecoration(labelText: 'Name'), keyboardType: TextInputType.name, ), ) ], ), Row( children: const [ Expanded( child: TextField( decoration: InputDecoration(labelText: 'Email'), keyboardType: TextInputType.emailAddress, ), ) ], ), Row( children: const [ Expanded( child: TextField( decoration: InputDecoration(labelText: 'Phone number'), keyboardType: TextInputType.phone, ), ) ], ), Row( children: const [ Expanded( child: TextField( decoration: InputDecoration(labelText: 'URL'), keyboardType: TextInputType.url, ), ) ], ), Row( children: const [ Expanded( child: TextField( decoration: InputDecoration(labelText: 'Datetime'), keyboardType: TextInputType.datetime, ), ) ], ), Row( children: const [ Expanded( child: TextField( decoration: InputDecoration(labelText: 'Number'), keyboardType: TextInputType.number, ), ) ], ), ], ), ), ), ); } }