TextField
has a lot of different configurations. Let’s discover how to set the color of the cursor.
TextField
has cursorColor
parameter.
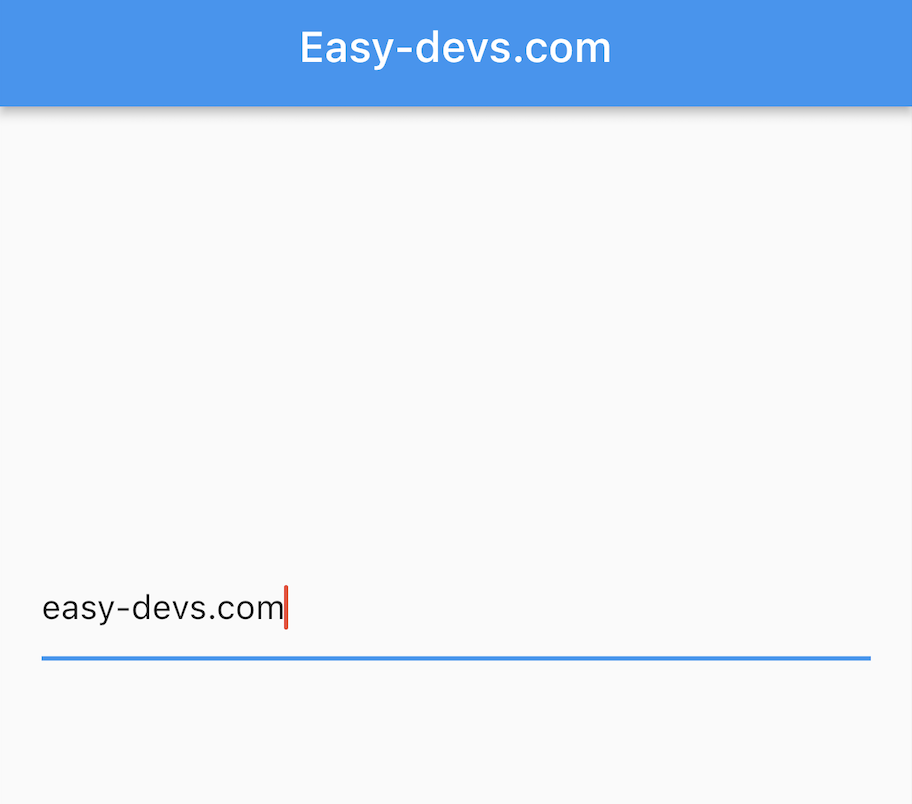
Full source code:
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( title: 'Easy-devs.com', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(title: 'Easy-devs.com'), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key, required this.title}); final String title; @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: const <Widget>[ Padding( padding: EdgeInsets.all(20.0), child: TextField( cursorColor: Colors.red, ), ) ], ), ), ); } }
The previous example shows you how to set the cursor color for the particular TextField. If you need to change it globally you can set in the ThemeData
.
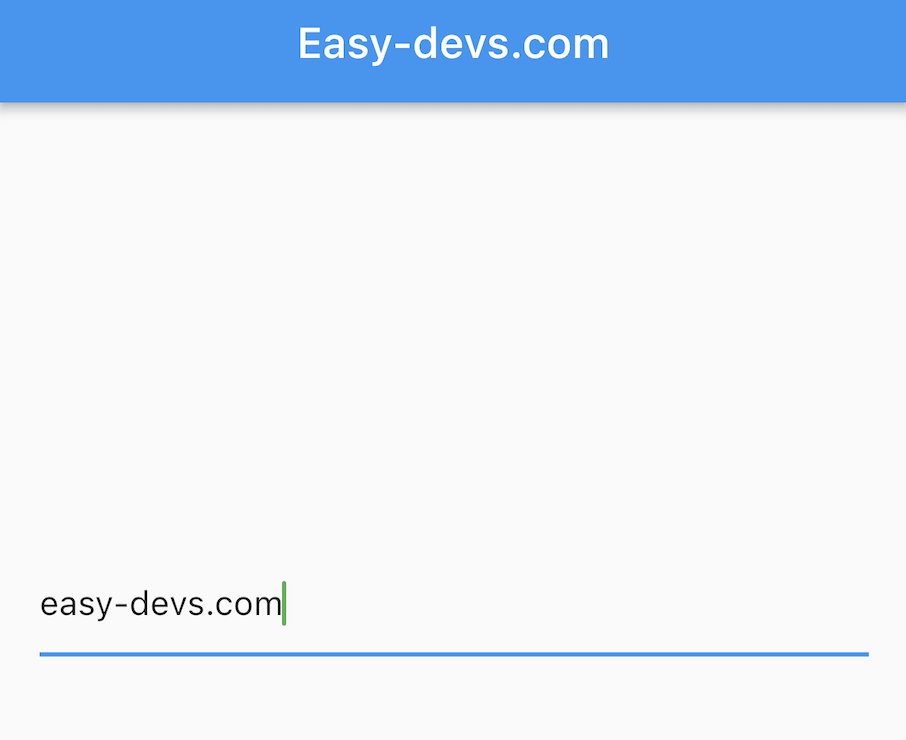
Full source code:
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( title: 'Easy-devs.com', theme: ThemeData( textSelectionTheme: const TextSelectionThemeData(cursorColor: Colors.green), primarySwatch: Colors.blue, ), home: const MyHomePage(title: 'Easy-devs.com'), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key, required this.title}); final String title; @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: const <Widget>[ Padding( padding: EdgeInsets.all(20.0), child: TextField(), ) ], ), ), ); } }